Spring MVC Application using Spring, Hibernate, Spring Security and Log4j.
- Admin
- May 2, 2017
- 5 min read
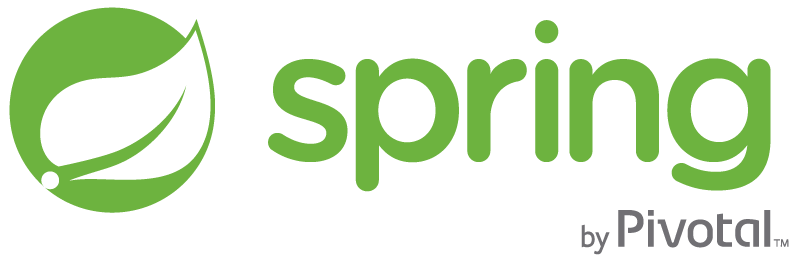
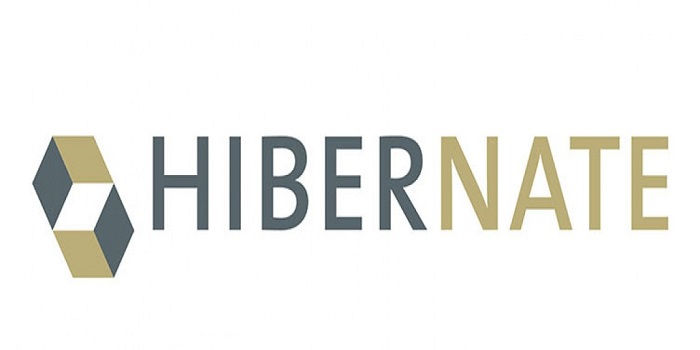
In this article we are going to create Shopping Portal application step by step using Spring MVC , hibernate and will apply Security using Spring- Security and integrate Log4j with our application for logging. We will use MySql database for storing our data.
Technologies in this article :
Maven 4.0.0
JDK 1.8
Hibernate 4.3.5.Final
Spring 4.2.5.RELEASE
Spring-Security 4.0.4.RELEASE
MySql 5.1.3
log4j 1.2.17
Demo
1. Create Maven Project In Eclipse
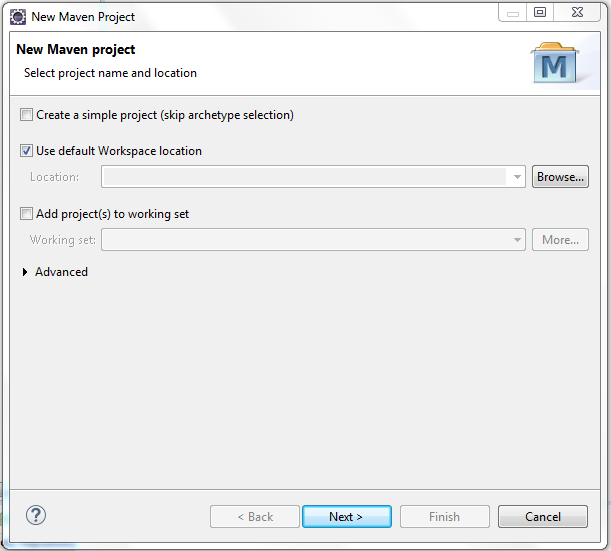
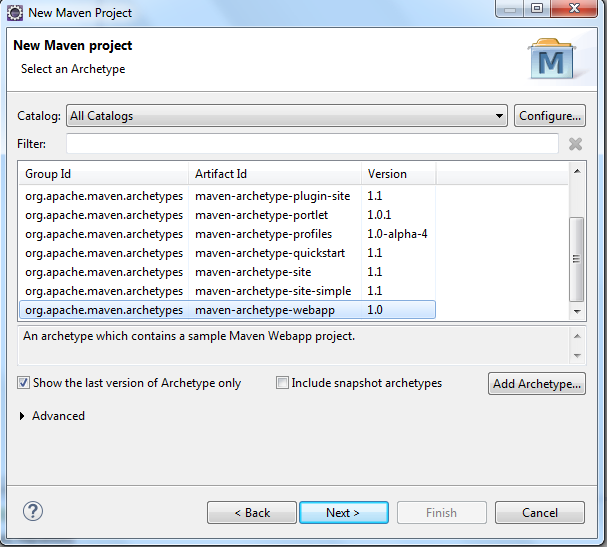
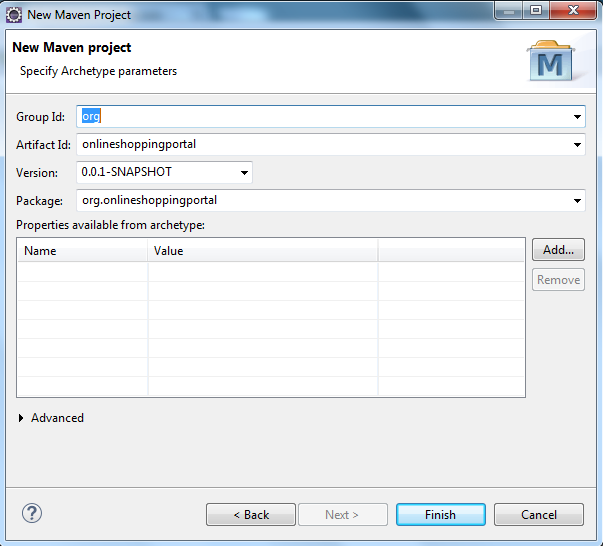
After finishing we will see Maven project structure in eclipse as shown below.

There should be three folder under src folder i.e main/java , main/resources and test/java. If they are not present than go to build path configuration than in java build path and select all checkbox and click apply.
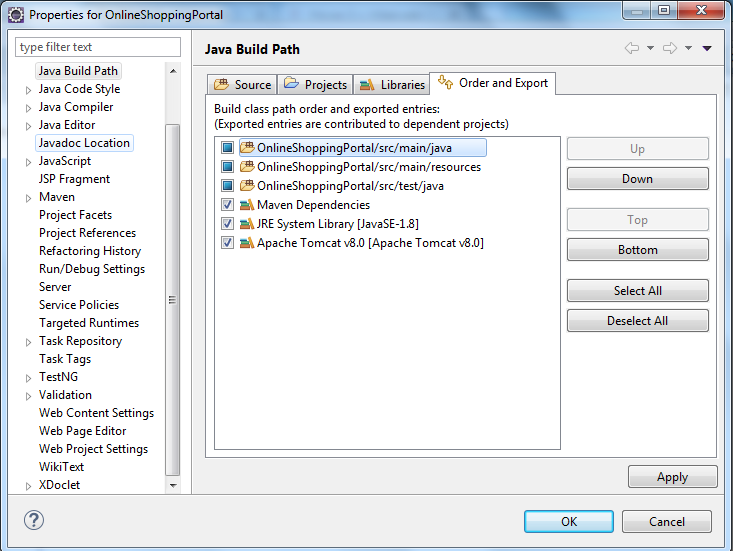
2. Add Dependencies.
File : pom.xml
<properties> <javax.servlet>3.1.0</javax.servlet> <javax.servlet.jsp>2.2</javax.servlet.jsp> <spring.version>4.2.5.RELEASE</spring.version> <spring.security.version>4.0.4.RELEASE</spring.security.version> <hibernate.version>4.3.5.Final</hibernate.version> <mysql.connector>5.1.34</mysql.connector> <commons.validator>1.5.0</commons.validator> <log4j.version>1.2.17</log4j.version> <jdk.version>1.8</jdk.version> <jstl>1.2</jstl> </properties>
<dependencies>
<!-- Log4j --> <dependency> <groupId>log4j</groupId> <artifactId>log4j</artifactId> <version>${log4j.version}</version> </dependency> <!-- http://mvnrepository.com/artifact/jstl/jstl --> <dependency> <groupId>jstl</groupId> <artifactId>jstl</artifactId> <version>${jstl}</version> </dependency> <!-- Servlet API --> <!-- http://mvnrepository.com/artifact/javax.servlet/javax.servlet-api --> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>${javax.servlet}</version> <scope>provided</scope> </dependency> <!-- JSP API --> <!-- http://mvnrepository.com/artifact/javax.servlet.jsp/jsp-api --> <dependency> <groupId>javax.servlet.jsp</groupId> <artifactId>jsp-api</artifactId> <version>${javax.servlet.jsp}</version> <scope>provided</scope> </dependency> <!-- Spring dependencies --> <!-- http://mvnrepository.com/artifact/org.springframework/spring-core --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>${spring.version}</version> </dependency> <!-- http://mvnrepository.com/artifact/org.springframework/spring-web --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-web</artifactId> <version>${spring.version}</version> </dependency> <!-- http://mvnrepository.com/artifact/org.springframework/spring-webmvc --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>${spring.version}</version> </dependency> <!-- http://mvnrepository.com/artifact/org.springframework/spring-orm --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-orm</artifactId> <version>${spring.version}</version> </dependency> <!-- Spring Security Artifacts - START -->
<!-- http://mvnrepository.com/artifact/org.springframework.security/spring-security-web --> <dependency> <groupId>org.springframework.security</groupId> <artifactId>spring-security-web</artifactId> <version>${spring.security.version}</version>
</dependency>
<!-- http://mvnrepository.com/artifact/org.springframework.security/spring-security-config -->
<dependency> <groupId>org.springframework.security</groupId> <artifactId>spring-security-config</artifactId> <version>${spring.security.version}</version> </dependency> <!-- http://mvnrepository.com/artifact/org.springframework.security/spring-security-taglibs --> <dependency> <groupId>org.springframework.security</groupId> <artifactId>spring-security-taglibs</artifactId> <version>${spring.security.version}</version>
</dependency> <!-- Hibernate --> <!-- http://mvnrepository.com/artifact/org.hibernate/hibernate-core --> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-core</artifactId> <version>${hibernate.version}</version> </dependency> <!-- http://mvnrepository.com/artifact/org.hibernate/hibernate-entitymanager --> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-entitymanager</artifactId> <version>${hibernate.version}</version> </dependency> <!-- http://mvnrepository.com/artifact/org.hibernate/hibernate-c3p0 -->
<dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-c3p0</artifactId> <version>${hibernate.version}</version> </dependency>
<!-- MySQL JDBC driver --> <!-- http://mvnrepository.com/artifact/mysql/mysql-connector-java --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>${mysql.connector}</version>
</dependency> <!-- Email validator,... --> <!-- http://mvnrepository.com/artifact/commons-validator/commons-validator --> <dependency> <groupId>commons-validator</groupId> <artifactId>commons-validator</artifactId> <version>${commons.validator}</version>
</dependency>
</dependencies>
3. Create Properties File For Data Source And Hibernate.
File : ds-hibernate-cfg.properties

Here we defined configuration properties for Data Source and for Hibernate.
For data source we defined driver class , url to the database schema , username and password of database.
For hibernate we defined
dialect = Dialect means "the variant of a language". Hibernate, as we know, is database agnostic. It can work with different databases. However, databases have proprietary extensions/native SQL variations, and set/sub-set of SQL standard implementations. Therefore at some point hibernate has to use database specific SQL. Hibernate uses "dialect" configuration to know which database you are using so that it can switch to the database specific SQL generator code wherever/whenever necessary.
show_sql = If true enable the logging of all the generated SQL statements to the console.
current_session_context_class = When you create a hibernate session using any of the sessionFactory.openSession(...) methods the session factory will 'bind' the session to the current context. The default context is 'thread' which means the sesion factory will bind the session to the thread from which openSession(...) is called. This is useful because you can later call sessionFactory.getCurrentSession() which will return the session that is bound to the currently running thread. You can use other predefined current_session_context_class values such as 'jta' which will bind the session to the currently running JTA transaction (if you're running in an application server that supports JTA this is really useful). Or you can write your own implementation of org.hibernate.context.CurrentSessionContext and use that implementation to manage the current session context (not really advisable unless you absolutely need to).
hbm2ddl.auto = hibernate.hbm2ddl.auto Automatically validates or exports schema DDL to the database when the SessionFactory is created. With create-drop, the database schema will be dropped when the SessionFactory is closed explicitly.e.g. validate | update | create | create-drop
So the list of possible options are,
validate: validate the schema, makes no changes to the database.
update: update the schema.
create: creates the schema, destroying previous data.
create-drop: drop the schema
when the SessionFactory is closed explicitly, typically when the application is stopped.
*Note : Keep this file direct under resources folder.
4. Create Message Properties File For Validating Forms.
File : validator.properties
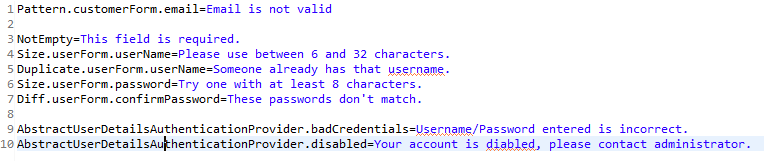
Here we defined properties for validating forms.
last two properties are defined by spring-security, we are overriding these properties here.
*Note : Keep this file under resources under messages folder.
5. Create Log4j Properties File.
File : log4j.properties
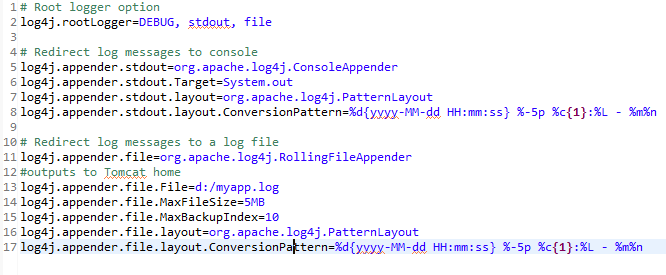
*Note : Keep this file direct under resources folder.
5. Create Configuration Files.
File : SpringWebAppInitializer.java

Interface WebApplicationInitializer = Interface to be implemented in Servlet 3.0+ environments in order to configure the ServletContext programmatically -- as opposed to (or possibly in conjunction with) the traditional web.xml-based approach.
File : ApplicationContextConfig.java

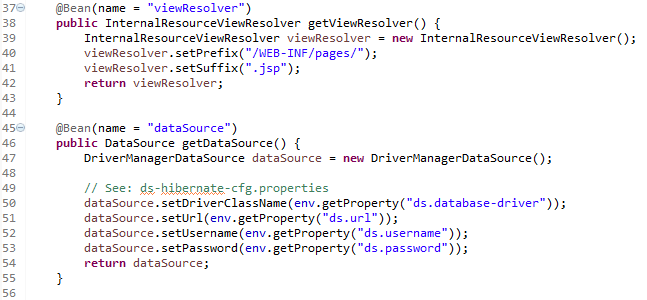

@ComponentScan = Component scanning with @ComponentScan is telling spring that it should search the class path for all the classes under org.onlineshopping.* and look at each class to see if it has a @Controller, or @Repository, or @Service, or @Component and if it does then Spring will register the class with the bean factory as if you had typed @Bean in the configuration class.
@EnableTransactionManagement = Enables Spring's annotation-driven transaction management capability, similar to the support found in Spring's <tx:*> XML namespace.
@PropertySource = @PropertySource annotation is used to externalize your configuration to a properties file and populate the Environment object with properties defined in properties files.
File : SpringSecurityInitializer.java

File : WebMvcConfig.java
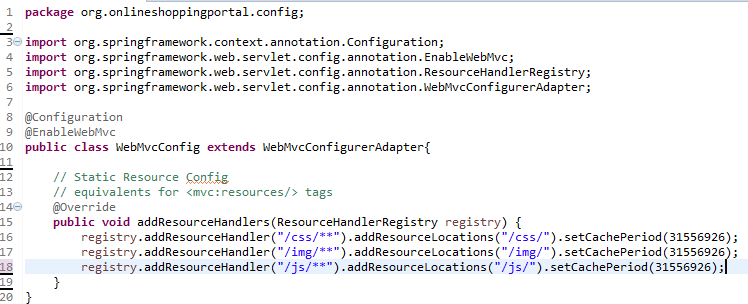
WebMvcConfig class enables Spring MVC with @EnableWebMvc annotation. It extends WebMvcConfigurerAdapter, which provides empty methods that can be overridden to customize default configuration of Spring MVC.
*Note : Create these files under org.onlineshoppingportal.config package.
6. Create Entity Classes.
File : Account.java
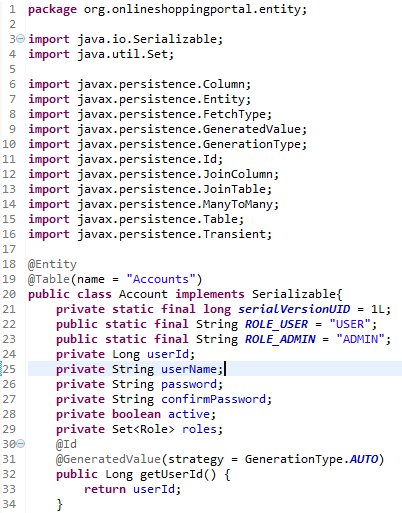
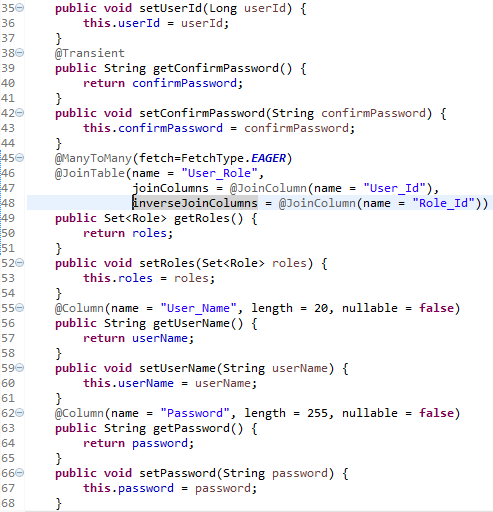
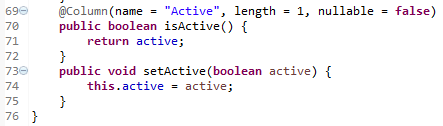
@Transient is used when we don't want to persist the corresponding field value in table.
File : Role.java
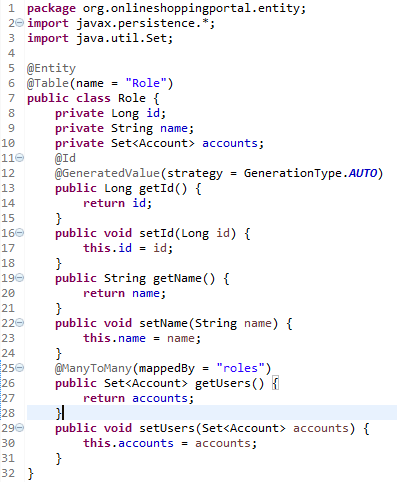
File : Product.java
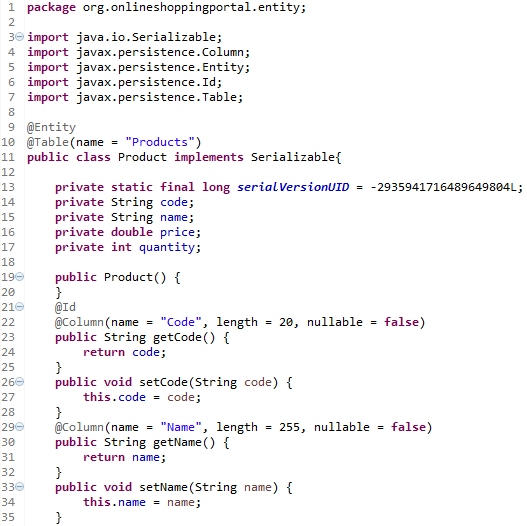

File : Order.java



*Note : Create these files under org.onlineshoppingportal.entity package.
7. Create DAO I/F and Implementation Classes.
File : AccountDao.java
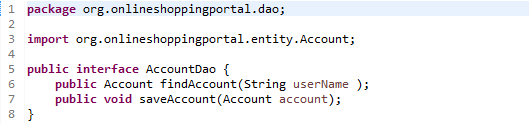
File : AccountDaoImpl.java
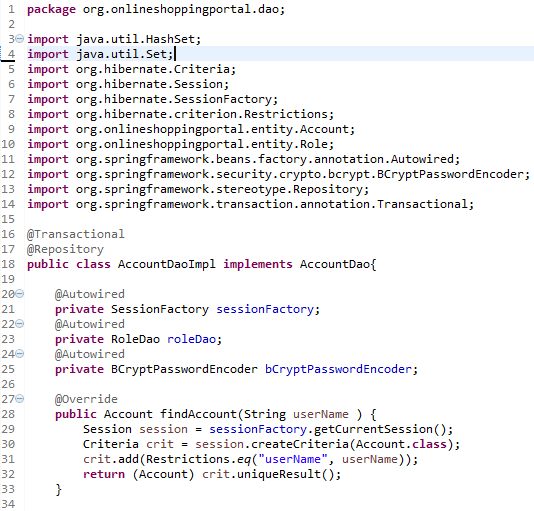
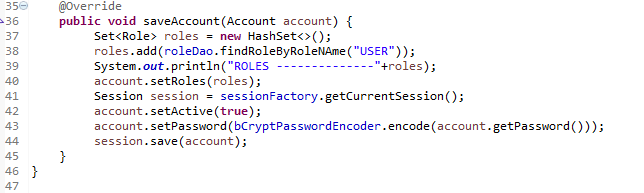
File : RoleDao.java
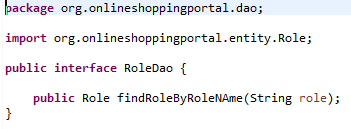
File : RoleDaoImpl.java
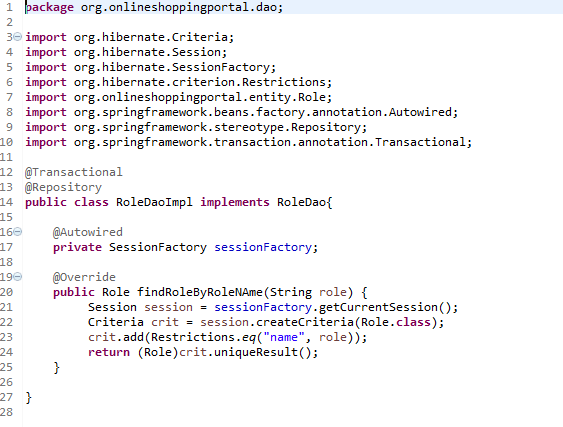
File : ProductDao.java
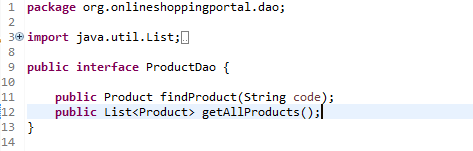
File : ProductDaoImpl.java
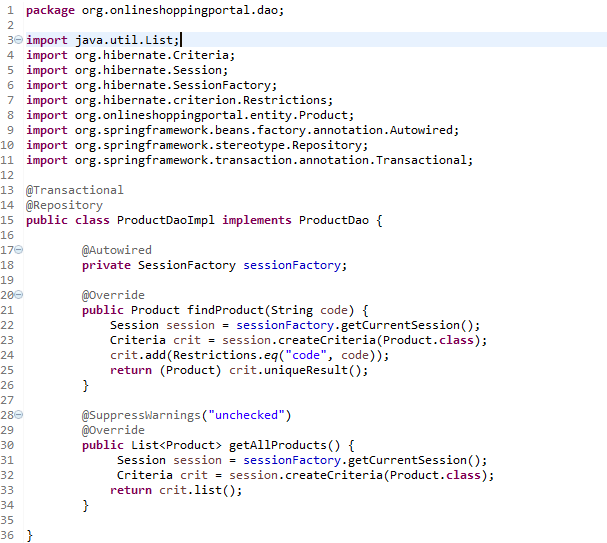
File : OrderDao.java
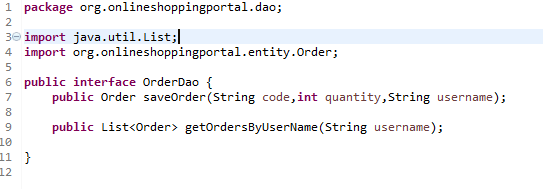
File : OrderDaoImpl.java
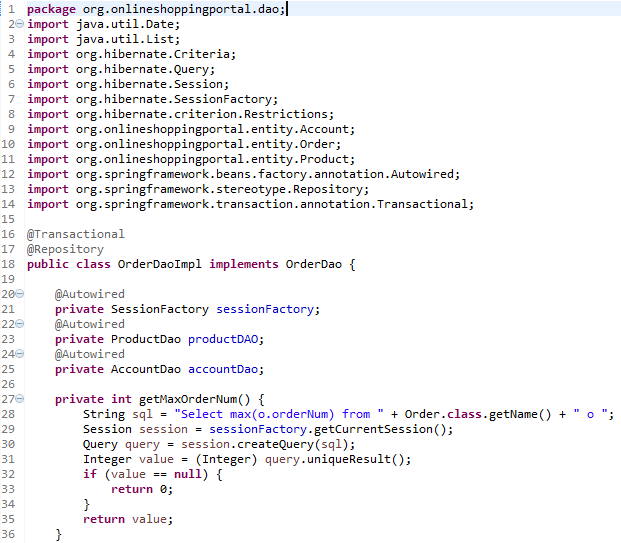
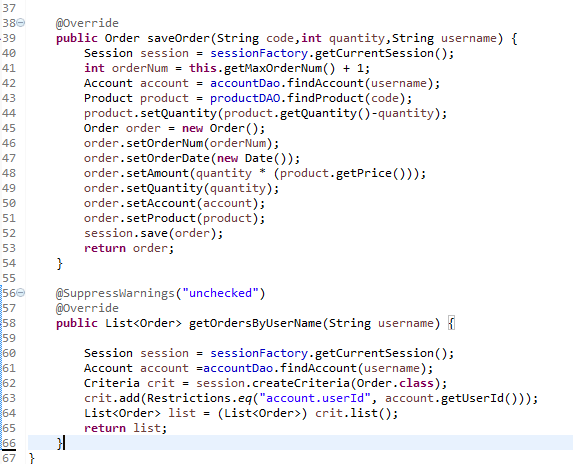
*Note : Create these files under org.onlineshoppingportal.dao package.
8. Create Validator Class.
File : AccountValidator.java

*Note : Create these files under org.onlineshoppingportal.validator package.
9. Create Custom Exception Classes.
File : AlreadyLoginException.java
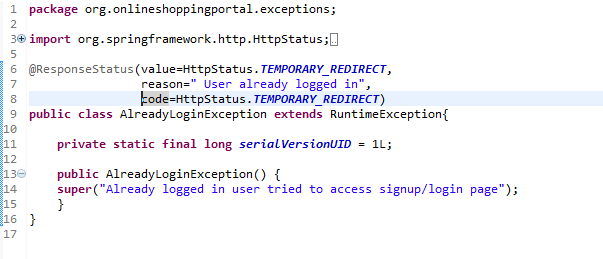
File : NoOrderFoundException.java
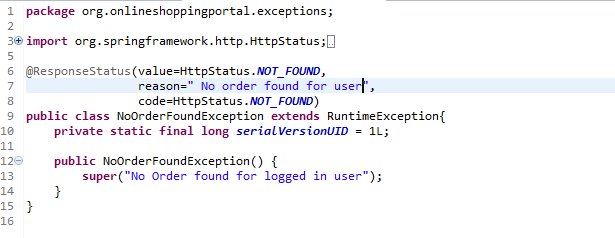
File : ProductOutOfStockException.java
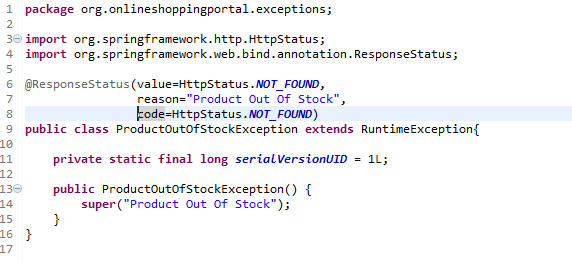
*Note : Create these files under org.onlineshoppingportal.exceptions package.
10. Create Authentication Service Classes.
File : MyDBAuthenticationService.java
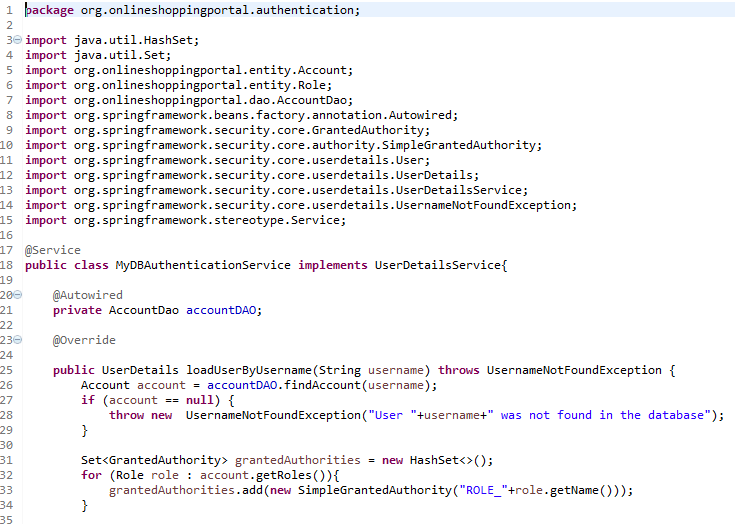
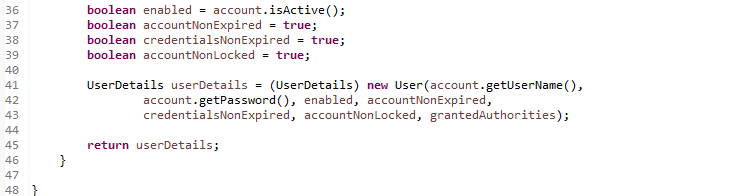
File : SecurityService.java
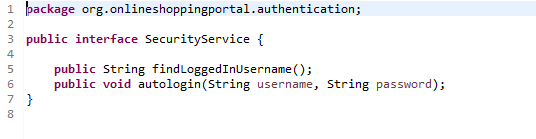
File : SecurityServiceImpl.java
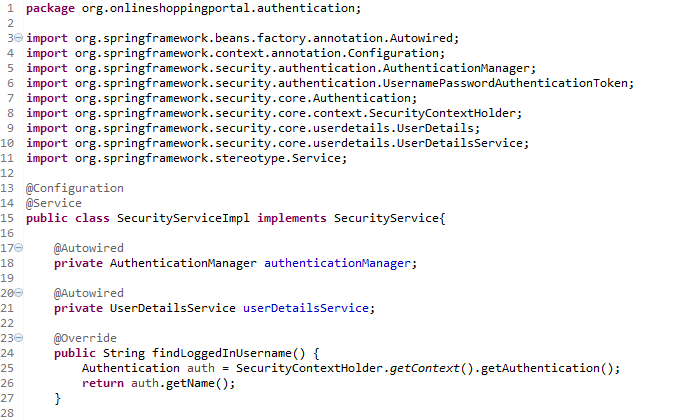

*Note : Create these files under org.onlineshoppingportal.authentication package.
11. Create Controller Classes.
File : MainController.java
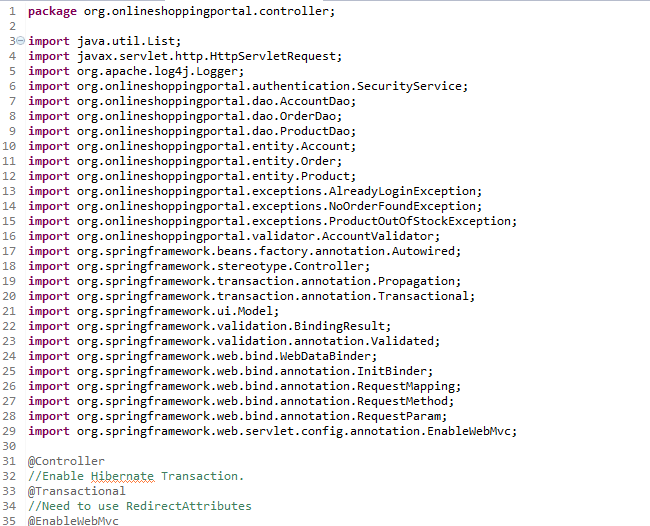
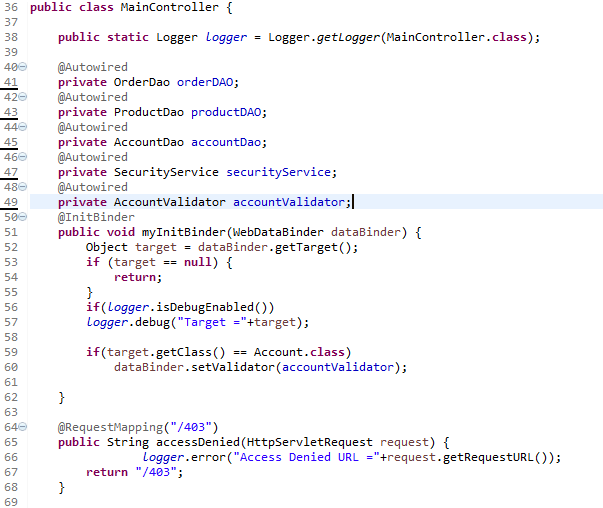
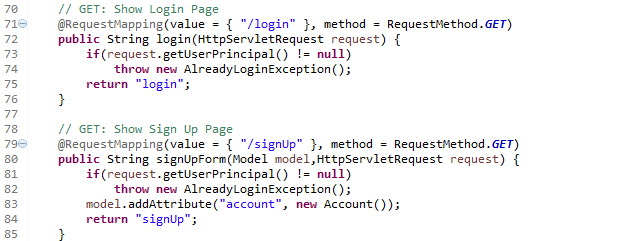
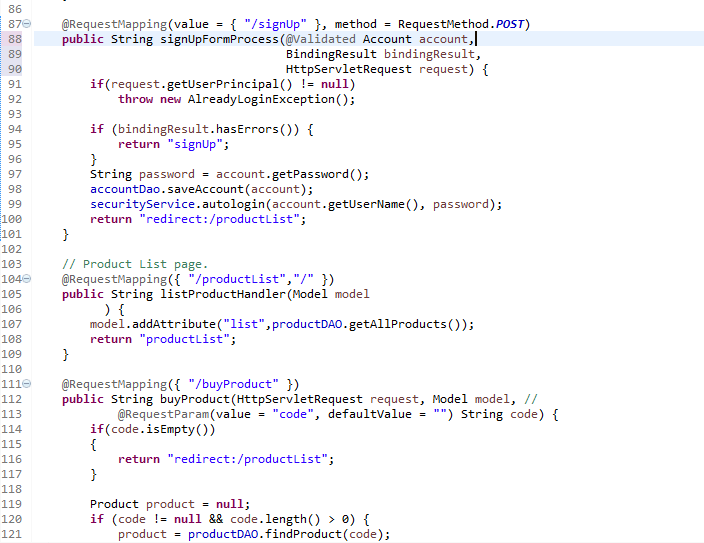
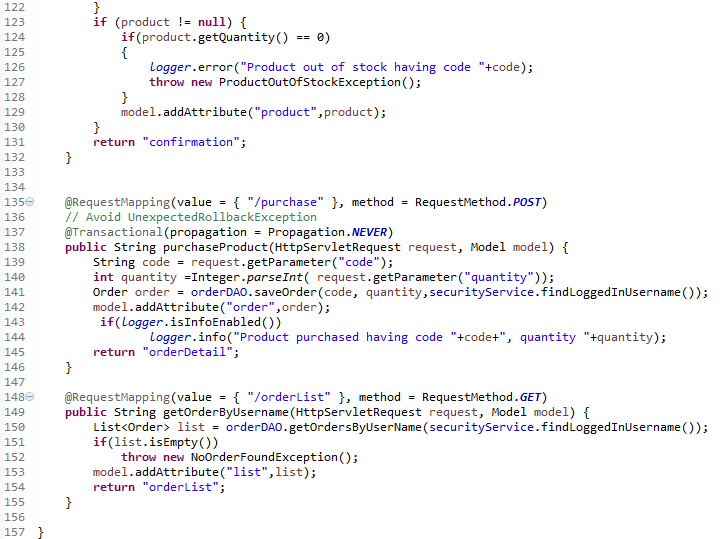
File : ExceptionController.java

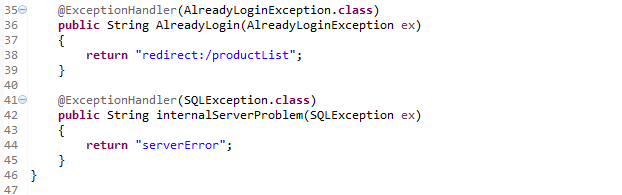
*Note : Create these files under org.onlineshoppingportal.controller package.
12. Create Web Security Configuration Class.
File : WebSecurityConfig.java
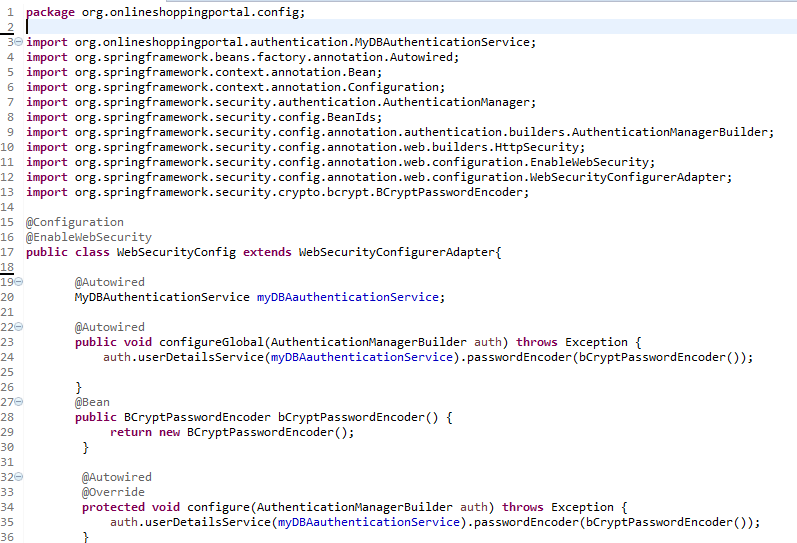
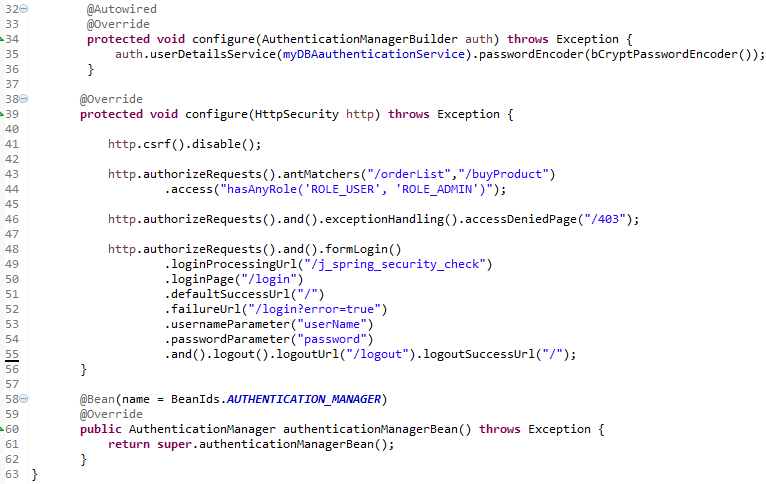
*Note : Create these files under org.onlineshoppingportal.config package.
13. Create JSP Files.
File : _header.jsp

File : _footer.jsp

File : _menu.jsp

File : login.jsp
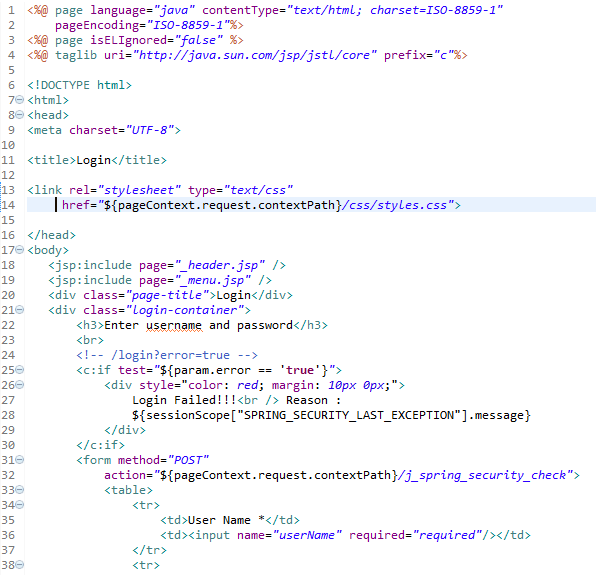

File : signup.jsp
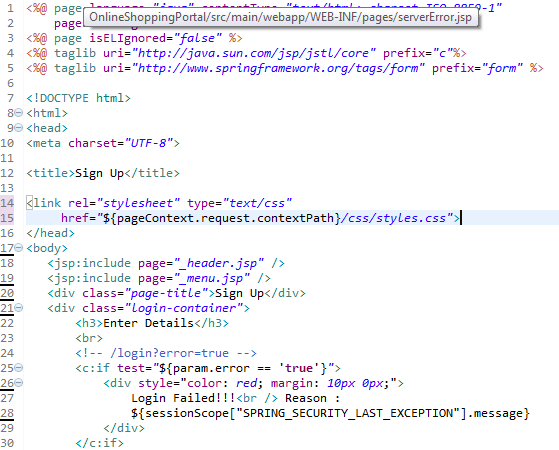
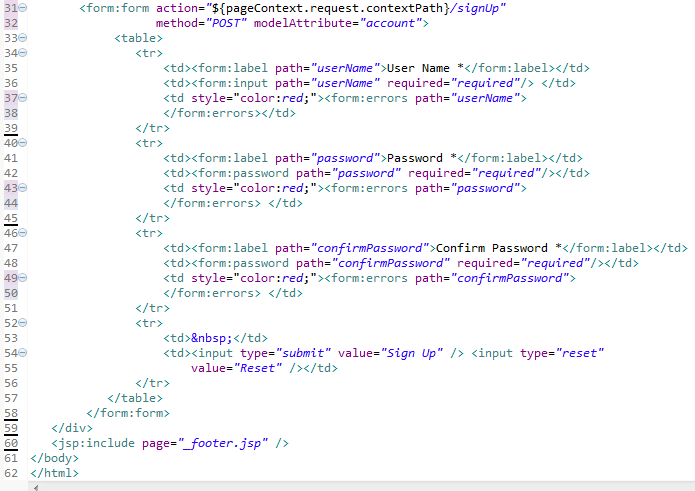
File : productList.jsp
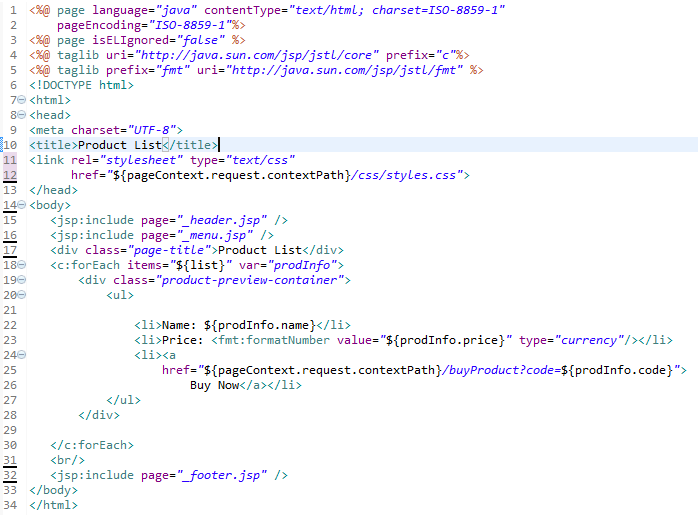
File : confirmation.jsp
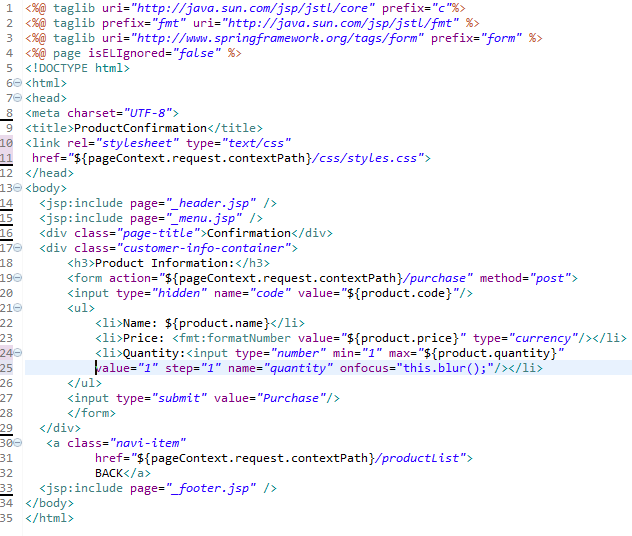
File : orderList.jsp
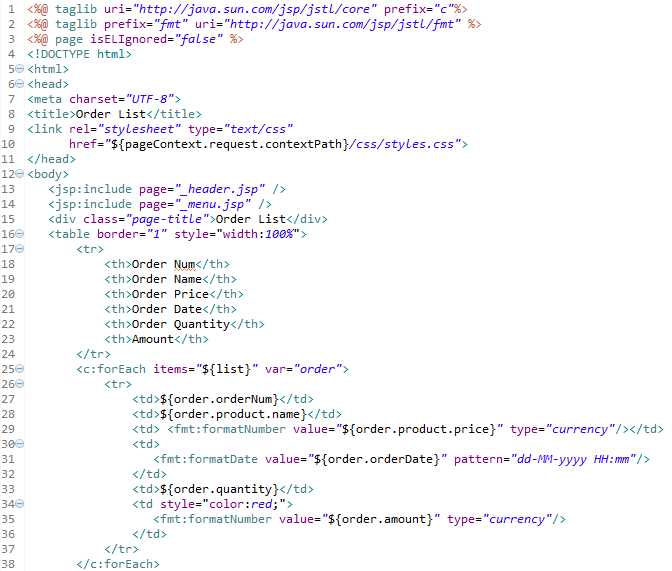

File : orderDetail.jsp

File : 403.jsp

File : 404.jsp
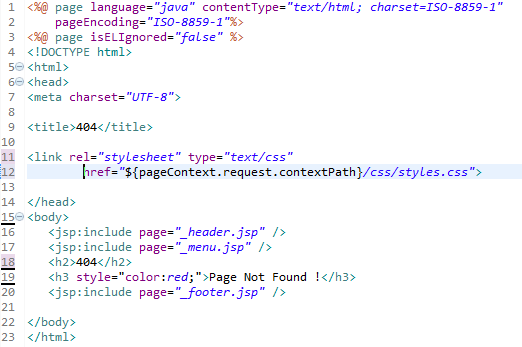
File : noOrder.jsp
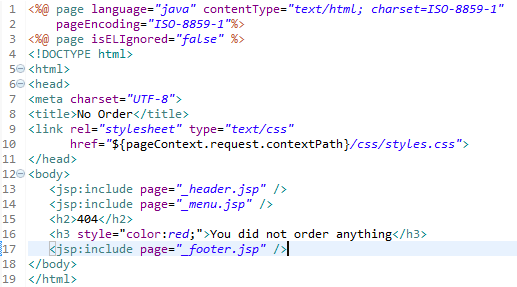
File : outOfStock.jsp
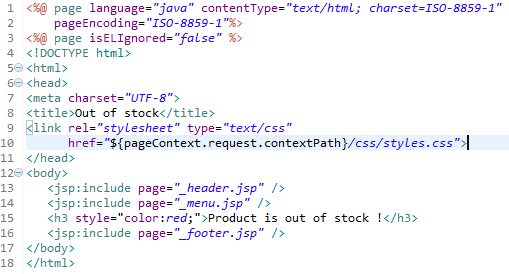
File : serverError.jsp
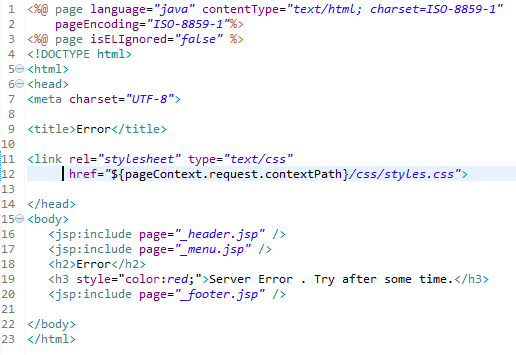
*Note : Create these files under webapp/WEB-INF/pages folder.
14. Create CSS File.
File : styles.css
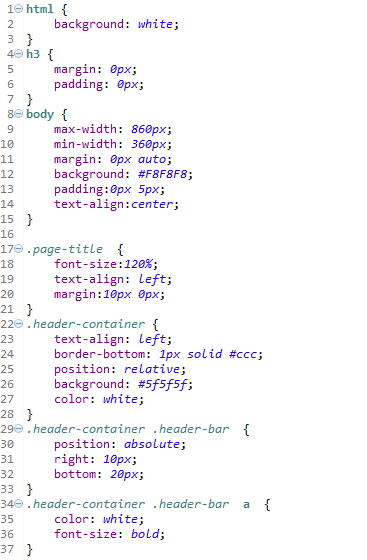
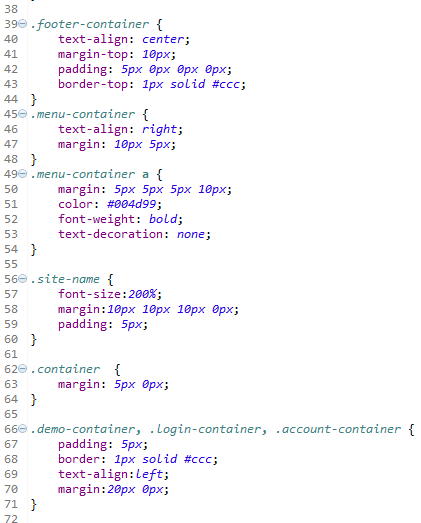
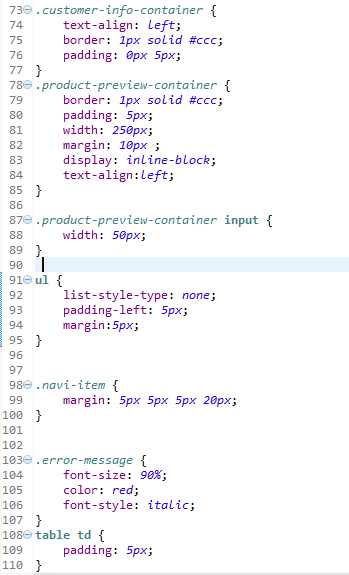
*Note : Create these files under webapp/css folder.
14. Project Structure.
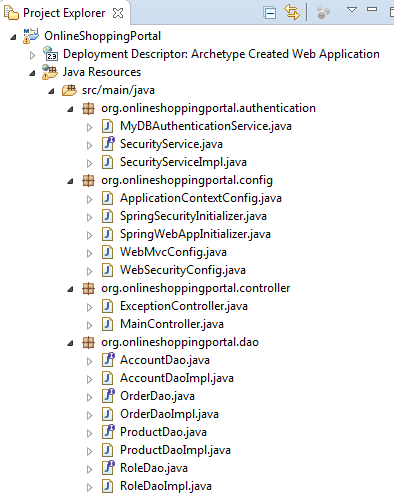

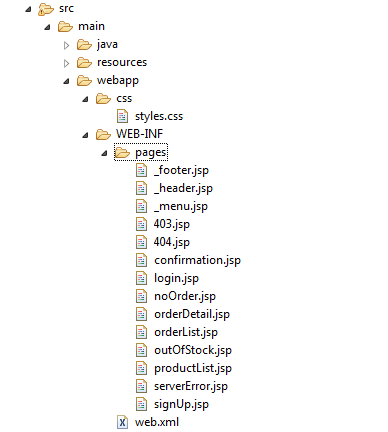
14. Steps To Do Before Running App.
Run these queries-
insert into role values(1,'USER'); insert into role values(2,'ADMIN');
insert into products values('S001','Java',150,20); insert into products values('S002','Java8',160,1); insert into products values('S003','node',170,0); insert into products values('S004','angular',180,2); insert into products values('S005','jsp',190,5); insert into products values('S006','spring',120,10); insert into products values('S007','hibernate',110,19);
Now the app is ready to run.
For Source Code GITHUB
Comments