Hibernate Configuration Without XML And Using XML Files.
- Admin
- May 5, 2017
- 3 min read
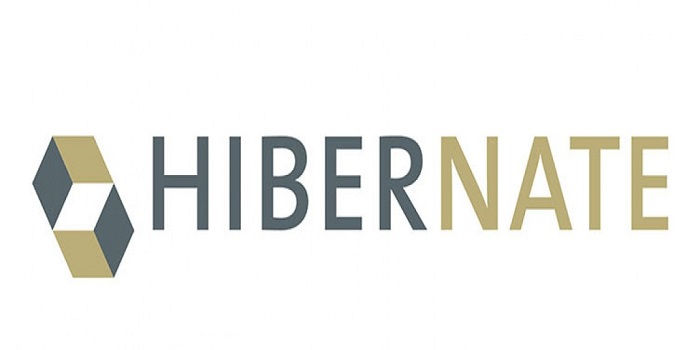
In this article we are going to configure hibernate using java class i.e. without XML file and using XML file.
We can configure hibernate using java class so no need to add hibernate.cfg.xml and can provide mapping of entities via annotated entity classes so no need to provide mapping files.
It is a handy and easy approach to configure hibernate.
Technologies in this article :
Maven 4.0.0
JDK 1.8
Hibernate 4.3.5.Final
1. Programmatic configuration
An instance of org.hibernate.cfg.Configuration represents an entire set of mappings of an application's Java types to an SQL database. The org.hibernate.cfg.Configuration is used to build an immutable org.hibernate.SessionFactory. The mappings are compiled from various XML mapping files.
You can obtain a org.hibernate.cfg.Configuration instance by instantiating it directly and specifying XML mapping documents. If the mapping files are in the classpath, use addResource(). For example:
Configuration cfg = new Configuration()
.addResource("Item.hbm.xml")
.addResource("Bid.hbm.xml");
An alternative way is to specify the mapped class and allow Hibernate to find the mapping document for you:
Configuration cfg = new Configuration()
.addClass(org.hibernate.auction.Item.class)
.addClass(org.hibernate.auction.Bid.class);
Hibernate will then search for mapping file named /org/hibernate/auction/Item.hbm.xml and /org/hibernate/auction/Bid.hbm.xml in the classpath. This approach eliminates any hardcoded filenames.
And if you defined annotated classes for mapping than no need to create mapping xml file.
For example:
Configuration cfg = new Configuration() .addAnnotatedClass(org.hibernate.auction.Item.class); .addAnnotatedClass(org.hibernate.auction.Bid.class);
A org.hibernate.cfg.Configuration also allows you to specify configuration properties. For example:
Configuration cfg = new Configuration()
.addClass(org.hibernate.auction.Item.class)
.addClass(org.hibernate.auction.Bid.class)
.setProperty("hibernate.dialect", "org.hibernate.dialect.MySQLInnoDBDialect") .setProperty("hibernate.connection.datasource", "java:comp/env/jdbc/test") .setProperty("hibernate.order_updates", "true");
1.1 Obtaining SessionFactory
In Hibernate, it was often to build a SessionFactory and pull out a Session as follows:
SessionFactory sessionFactory = new Configuration().configure().buildSessionFactory();
Session session = sessionFactory.openSession();
However, since Hibernate 4.x, this approach is deprecated. According to Hibernate 4.0 API docs, the Configuration class buildSessionFactory() method is deprecated and it recommends developers to use the buildSessionFactory(ServiceRegistry) instead. Here is the new recommended code snippet that builds the SessionFactory based on a ServiceRegistry and obtains the Session:
Configuration configuration = new Configuration().configure();
ServiceRegistryBuilder registry = new ServiceRegistryBuilder();
registry.applySettings(configuration.getProperties());
ServiceRegistry serviceRegistry = registry.buildServiceRegistry();
SessionFactory sessionFactory = configuration.buildSessionFactory(serviceRegistry);
Session session = sessionFactory.openSession();
The Configuration class’ configure() method loads mappings and properties from the convention resource file hibernate.cfg.xml which should be present in the classpath.
Note * - If you are not using hibernate.cfg.xml file and doing configuration programmatic than don't call configure method of Configuration class. If you call configure method than hibernate will search for hibernate.cfg.xml file and if not found than it will throw exception.
However, since hibernate 4.3 ServiceRegisteryBuilder also get deprecated and in place of this StandardServiceRegistryBuilder is used to create ServiceRegistry.
Properties prop = new Properties() .setProperty("connection.driver_class", "com.mysql.jdbc.Driver") .setProperty("hibernate.connection.url", "jdbc:mysql://localhost:3306/xyz") .setProperty("hibernate.connection.username", "root") .setProperty("hibernate.connection.password", "root") .setProperty("hibernate.dialect", "org.hibernate.dialect.MySQLDialect"); Configuration cfg = new Configuration() .addAnnotatedClass(org.entity.StudentDetails.class) .addAnnotatedClass(org.entity.Student.class) .setProperties(prop); ServiceRegistry serviceRegistry = new StandardServiceRegistryBuilder() .applySettings(cfg.getProperties()).build(); SessionFactory sf = buildSessionFactory(serviceRegistry);
So it is better if we will create HibernateUtill class to provide our SessionFactory which we are going to use to obtain a Session.
HibernateUtill.java

Note*- This Utill class is compatible with version above 4.3 .
2. XML Configuration
Create Hibernate config file named hibernate.cfg.xml and put it in classpath(under resource folder).
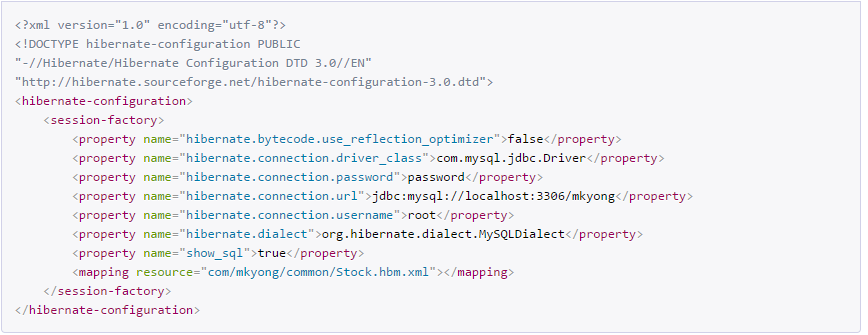
Now create hibernate utill class to load this file.
Note* : If you are providing mapping via class not via xml file (*.hbm.xml) than you have to use <mapping class="org.entity.student"/> in place of
<mapping resource="student.hbm.xml" />
2.1 Obtaining SessionFactory
File: HibernateUtil.java

File: HibernateUtil.java (For hibernate version above 4.1,4.2 and 4.3)

However, since Hibernate 4.x, this approach is deprecated.
File: HibernateUtil.java (For hibernate version above 4.3)
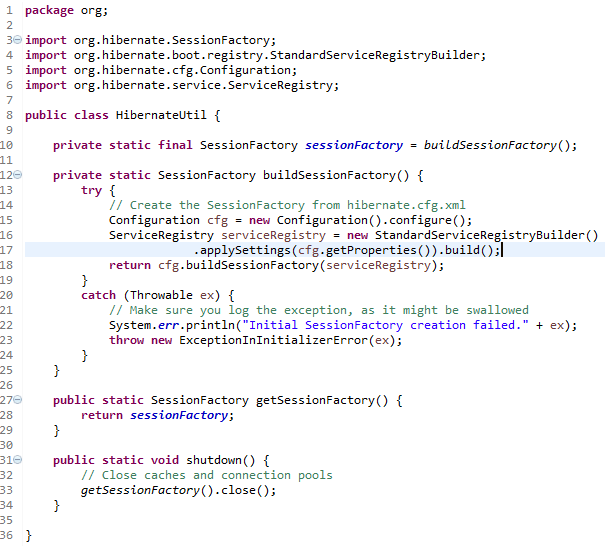
However, since hibernate 4.3 ServiceRegisteryBuilder also get deprecated and in place of this StandardServiceRegistryBuilder is used to create ServiceRegistry.
Note* : It is not necessary to named your file as hibernate.cfg.xml, If you named your file other than hibernate.cfg.xml than you have to supply your name in cofigure(String resourceName) method as argument and it is not necessary to keep your file under classpath , if you kept your file under any other folder than you have to create file object and provide it to configure(File file) method or provide url in configure(Url url) method.
If you do not provide any argument to configure() method than hibernate by default search for file named hibernate.cfg.xml under resource path.
3. Hibernate Properties.
We can define various properties for hibernate which are useful for us.Some properties are as follows -


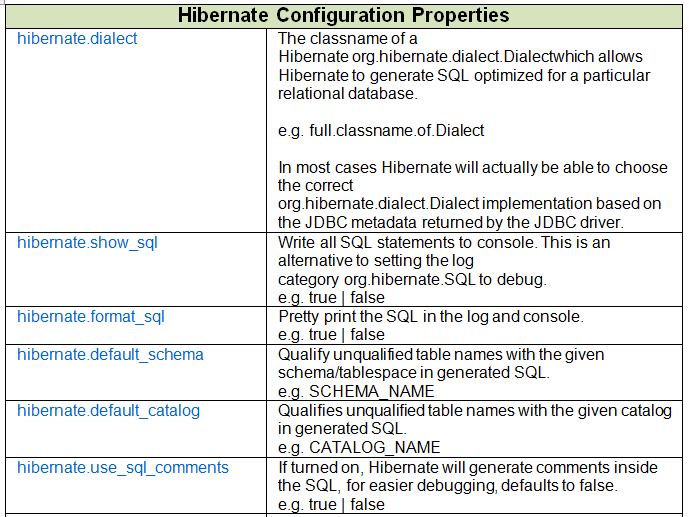

So using these properties name and their corresponding values we can configure hibernate.There are other properties also but we are showing only those properties which are used often in initial learning.
Hibernate One To One relationship using annotations see our Next Post
Don,t forget to like post and feel free to post yours queries in comment section.
Comments