Hibernate One To One Relationship Using Annotations (Unidirectional).
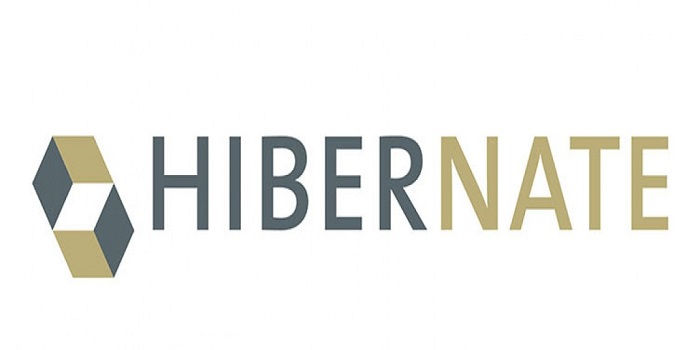
In this article we are going to explain one to one relationship in hibernate using annotations and will going to explain various annotations used and their effects on tables structure.
Technologies used in this article :
Maven 4.0.0
JDK 1.8
Hibernate 4.3.5.Final
MySql 5.1.34
In this example you will learn how to map one-to-one relationship using Hibernate. Consider the following relationship between Student and StudentDeatails entity.

According to the relationship each student should have a unique StudentDetails.
There are two types of relationship one is "unidirectional" and another is "bidirectional".
The term “bidirectional” literally means “functioning in two directions”, which is the concept that we will apply in our relationships between two Java objects. When we have a bidirectional relationship between objects, it means that we are able to access Object A from Object B, and Object B from Object A. Also it allows you to apply cascading options to both directions.
What is cascading and how to achieve bidirectional properties we will see in our example.
1. Project Structure.
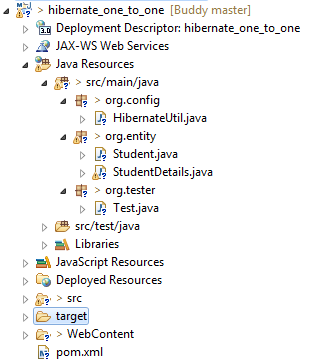
2. Dependencies.
File : pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org</groupId> <artifactId>hibernate_one_to_one</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging>
<name>hibernate_one_to_one</name> <url>http://maven.apache.org</url>
<properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <hibernate.version>4.3.5.Final</hibernate.version> <jdk.version>1.8</jdk.version> <mysql.connector>5.1.34</mysql.connector> </properties>
<dependencies> <!-- Hibernate --> <!-- http://mvnrepository.com/artifact/org.hibernate/hibernate-core --> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-core</artifactId> <version>${hibernate.version}</version> </dependency> <!-- http://mvnrepository.com/artifact/org.hibernate/hibernate-entitymanager --> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-entitymanager</artifactId> <version>${hibernate.version}</version> </dependency> <!-- http://mvnrepository.com/artifact/org.hibernate/hibernate-c3p0 --> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-c3p0</artifactId> <version>${hibernate.version}</version> </dependency> <!-- MySQL JDBC driver --> <!-- http://mvnrepository.com/artifact/mysql/mysql-connector-java --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>${mysql.connector}</version> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.1</version> <configuration> <source>1.8</source> <target>1.8</target> </configuration> </plugin> </plugins> </build> </project>
3. Hibernate Configuration.
Before start mapping between our entity classes we have to configure hibernate. For hibernate configuration related info please refer to our previous post Hibernate Configuration Without XML And Using XML Files.
File : HibernateUtil.java
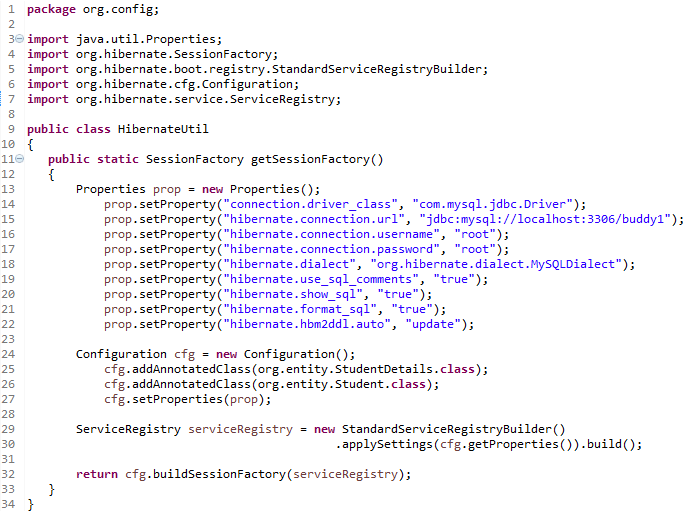
4. Hibernate One To One Relationship Unidirectional.
"Unidirectional" means "functioning in only one direction", which means we are able to access Object B from Object A but can't be able to access Object A from Object B. Also it allows you to apply cascading options in only one directions from owner entity to child entity.
In our case Object A is Student class which owes ownership.
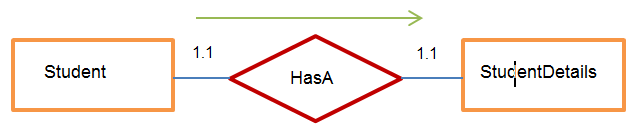
Above diagram shows one to one relationship and relationship lies from Student to StudentDetails in unidirectional which means Student class own the relationship.
4.1 Entity Classes.
File : Student.java
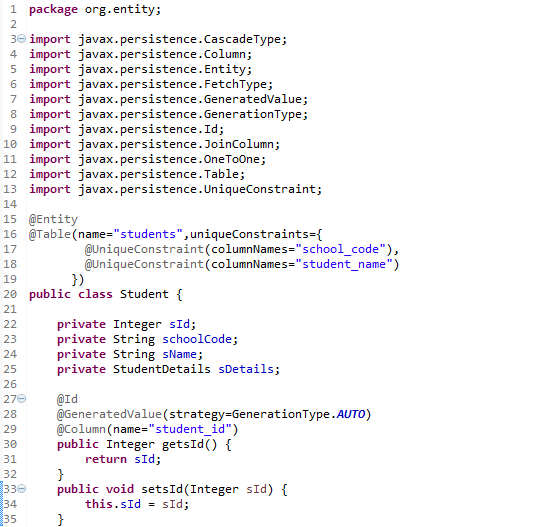

File : StudentDetails.java
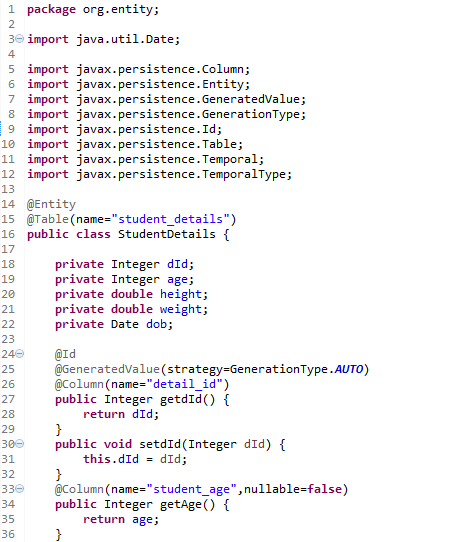
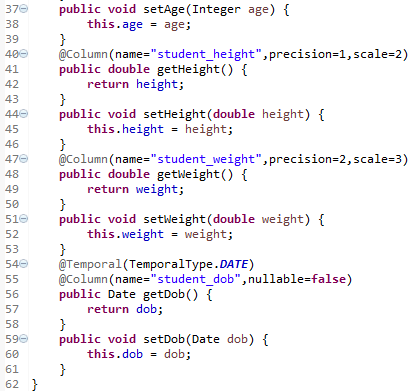
@Entity Annotation:
The @Entity annotation is a class level annotation and is Mandatory to use.It is used to mark respective class as entity bean, so class must have a no-argument constructor that is visible with at least protected scope.
@Table Annotation:
The @Table annotation is a class level annotation , it allows you to specify the details of the table that will be used to persist the entity in the database.
The @Table provides four attributes, allowing you to override the name of the table, its catalogue, and its schema, and enforce unique constraints on columns in the table.
@Id and @GeneratedValue Annotation:
The @Id annotation is method level or field level annotation. We can use this annotation on field level or on getter method of corresponding field.Each entity bean will have a primary key, which you annotate on the class with the @Id annotation.The primary can be single field or combination of multiple fields depending on the scenario.
By default , the @Id annotation will automatically determine the most appropriate primary key generation strategy to be used but you can override this by applying the @GenratedValue annotation which takes two parameters strategy and generation.
Note*: We will discuss what is strategy and generation in upcoming post.Please subscribe to our site to get notification for upcoming posts.
@Column Annotation:
The @Column annotation is field level or method level annotation. It is used to specify the details of the column to which a field or property will be mapped. it has following attributes:
name attribute permits the name of the column to be explicitly specified.
length attribute permits the size of the column, used for string value.
nullable attribute permits the column to be marked NOT NULL.
unique attribute permits the column to be marked as containing only unique values.
precision attribute permits the column precision value. the precision for a decimal (exact numeric) column. (Applies only if a decimal column is used.)
scale attribute defines the scale for a decimal (exact numeric) column. (Applies only if a decimal column is used.)
@Temporal Annotation:
The @Temporal annotation in JPA implementation can only be used with the fields and property get methods. Also, this annotation can be specified for the persistent fields or properties java.util.Data or java.util.Calendar. This annotation is available since the release of JPA 1.0. @Temporal solves the one of the major issue of converting the date and time values from Java object to compatible database type and retrieving back to the application. When Java class declares the fields java.sql.Date or java.sql.Time, then it is compatible with the database types and will not have any issues. But, when we are using the java.util.Date or java.util.Calendar, then we have to specify Temporal types to map the appropriate database types when persisting to the database.
@OneToOne Annotation:
The @OneToOne annotation is field level or method level annotation. It defines a single-valued association to another entity that has one-to-one multiplicity.It has following attributes:
fetch attribute defines the fetching strategy whether it is lazy or eager.
cascade attribute is use to cascade the operation performed on owner entity to child entity. it means if you perform insert, update, delete etc operation on owner entity than it will going to automatically perform on child class also.In one to one relationship it is by always eager whether you define it as eager or lazy.
orphanRemoval defines whether to apply the remove operation to entities that have been removed from the relationship and to cascade the remove operation to those entities.
@JoinColumn Annotation:
The @JoinColumn annotation is field level or method level annotation. It is used to define the properties for join column.
Note : join column is used to map the two entities.
4.2 Tester Classes:
File : SaveTester.java
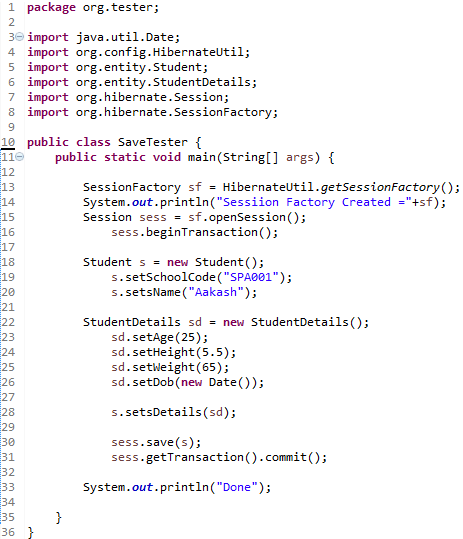
Output :
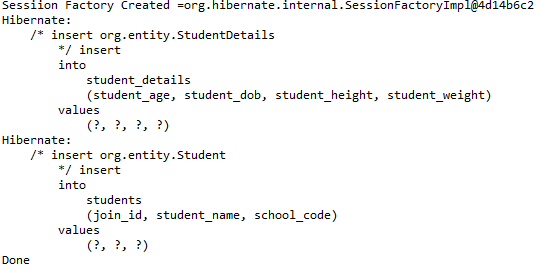
Table Structure:
students table

student_details table

Note*: Join column is present in owner table which is students table.
File : UpdateTester.java
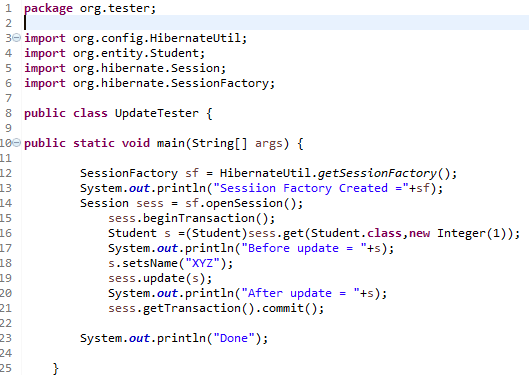
Output :

Note**: As you can see in program we update user first and than we print the updated user information on console but in output we see that updated user information first get printed than updated query fired.
It is because the updated query only fired when we commit transaction , in between from opening the transaction to commit the transaction changes done on student only reflects in cache. so when transaction is committed only than changes updated in database.
File : DeleteTester.java
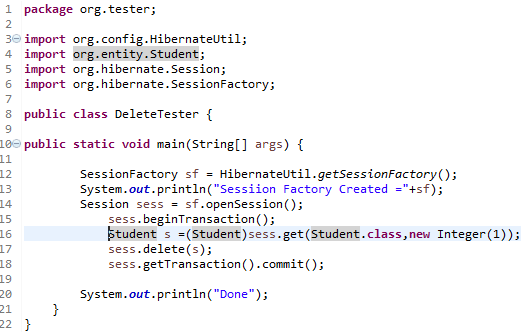
Output :
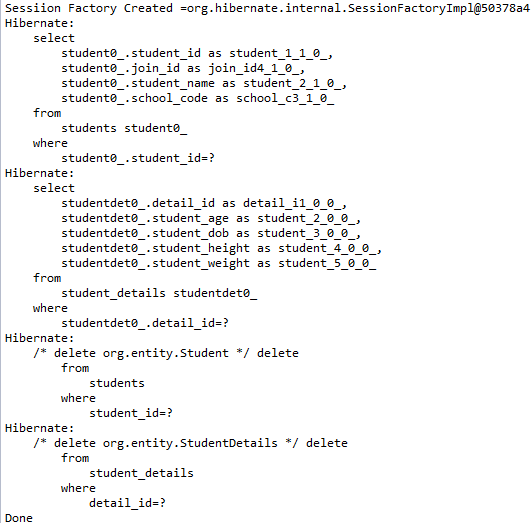
Note*: When you delete owner class object than its corresponding child class object also get deleted.
Find related source code on GITHUB.
In next post we explained Hibernate One To One relationship (Bidirectional) and mappedBy attribute of @OneToOne annotation.
For updates of our next post please subscribe below and don't forget to like. In case of any queries write in comment section.
Comments